2D Ising Model#
FIZ371 - Scientific & Technical Computations | 10/06/2020
2D Ising Model
Introduction
Relevance of Ising models
Ferromagnetic Materials
Difficulties
Monte Carlo simulation
Metropolis algorithm
Implementation
Homework
Appendix: Evolution of the configuration probabilities for a 2x2 Ising Grid
External link(s)
Dr. Emre S. Tasci emre.tasci@hacettepe.edu.tr
Introduction#
The Ising model is the modelling of a network with nodes interacting with each other with respect to some order. Initially developed by Ernst Ising in 1925 to model the phase transition in 1D in order to observe critical variables in action, alas Ising didn’t manage to encounter any phase transition and gave up the model, until much later. The model was analytically solved by Lars Onsager in 1944. The 3D version has not yet been solved although there are a couple of claims.
Relevance of Ising models#
As for the relevance and importance of Ising models, I’m quoting directly from our textbook, MacKay’s “Information Theory, Inference, and Learning Algorithms”:
Ising models are relevant for three reasons.
Ising models are important first as models of magnetic systems that have a phase transition. The theory of universality in statistical physics shows that all systems with the same dimension (here, two), and the same symmetries, have equivalent critical properties, i.e., the scaling laws shown by their phase transitions are identical. So by studying Ising models we can find out not only about magnetic phase transitions but also about phase transitions in many other systems.
Second, if we generalize the energy function to
where the couplings \(J_{mn}\) and applied fields \(h_n\) are not constant, we obtain a family of models known as ‘spin glasses’ to physicists, and as ‘Hopfield networks’ or ‘Boltzmann machines’ to the neural network community. In some of these models, all spins are declared to be neighbours of each other, in which case physicists call the system an “infinite-range” spin glass, and networks call it a “fully connected” network.
Third, the Ising model is also useful as a statistical model on its own right.
Ferromagnetic Materials#
A ferromagnetic material is a material whose atomic spins align with the applied external magnetic field and even at the lack of an external field, its atoms also tend to align their magnetic moments with that of their neighbours resulting in spin domains:
The magnetic contribution to the energy comes from the following interaction in the Hamiltonian:
Here, \(\vec{x}\) is the spin configuration of the sites; \(x_i\) is the spin of the ith site (-1 for “down” and +1 for “up”); \(J_{mn}\) is the coupling coefficient between the \(m\) and \(n\) sites and \(H\) is the applied external field, if there is any.
We can define the interaction depth of the spins via the coupling coefficient \(J_{mn}\): for example, the coefficient can be 5 for the nearest neighbours, 3 for the next nearest neighbours and 0 for the rest of the atoms making them irrelevant to the site at hand. J’s sign indicates the ferromagnetic/antiferromagnetic tendency, with \(J>0\) the spins tend to align in the same direction whereas negative \(J\) drives the antiferromagnetic behaviour.
For simplicity, we will assume \(J_{mn} = J\) if \(m\) and \(n\) are neighbours and \(J_{mn} = 0\) otherwise, so the only manipulation to the spins will be due to the neighbours.
As we have the energy equation, at equilibrium at temperature \(T\), the probability that the state is \(\vec{x}\), will be given by:
here, \(\beta=1/k_B T\), \(k_B\) is Boltzmann’s contant and:
is the partition function. From statistics, we know that the entropy can be written as:
where \(F\) is the free energy:
Difficulties#
So, from the previous section, as we have the probability equation, we can use the partition function \(Z\) to first to calculate the free energy and then the entropy of our system. However, there is a very bit tiny problem with the calculation of the partition function.
Even with a very small system of \(10\times10\) grid, where each site can have a sping value of \(\pm1\), we will have \(2^{100}\) possible configurations (\(\{\vec x\}\)), which will take more than the age of the universe to calculate on a supercomputer as we know it.
In contrast to this, calculating the energy of any given configuration \(\vec{x}\) is pretty much straightforward and fast, so we need to be able to exploit this fact for sampling.
Monte Carlo simulation#
Metropolis algorithm#
We will employ the Metropolis algorithm (which we have already considered as one of the stochastic activation functions of the artificial neural networks) and will randomly choose a site whose spin is in the state \(x_n\). If the spin is flipped, the change in the energy will be equal to:
where \(b_n\) is the local field, that is:
As the Metropolis algorithm proposes, if the energy is decreased, we automatically accept this spin flip; else, we still accept it with a probability of \(\exp(-\beta \Delta E)\):
After sufficient number of iterations, this method converges to the equilibrium distribution.
Implementation#
The following code is more suitable for an environment other than Jupyter as it produces many output plots.
#!/usr/bin/env python3
# -*- coding: utf-8 -*-
"""
Implementation of the Metropolis algorithm
for 2D Ising model evolution.
Written for the FIZ371 Course, 10/06/2020
Dr. Emre S. Tasci <emre.tasci@hacettepe.edu.tr>
"""
import numpy as np
import matplotlib.pyplot as plt
import matplotlib as mpl
# mpl.use('module://backend_interagg') # PyCharm doesn't always display the plots
colors = ['yellow', 'purple', 'orange', 'blue', 'yellow', 'purple']
bounds = [-1,1,2,3,4,5,6]
# Up: Purple | Down: Yellow
cmap = mpl.colors.ListedColormap(colors)
norm = mpl.colors.BoundaryNorm(bounds, cmap.N)
np.random.seed(371)
N = 10
num_iterations = 10000
H = 0 # External field
k_B = 1 # Boltzmann constant
J = 1 # Coupling coefficient
T0 = 10 # Temperature
beta = 1/(k_B*T0)
# Create our NxN grid, fill with random spin orientation
mapN = np.random.randint(0,2,[N,N])
#mapN = np.zeros((N,N))
mapN[mapN==0] = -1
print(mapN)
num_ups = np.sum(np.sum(mapN==1))
num_downs = N**2 - num_ups
print("{:} up | {:} down ".format(num_ups,num_downs))
plt.matshow(mapN, interpolation="none", cmap=cmap, norm=norm)
#plt.savefig("/tmp/figs/" + "TS_%.3fs" % (T0) + ".png")
plt.show()
#input("devam?..")
magnetization_vs_T = np.empty([0,2])
print("="*45)
for T in np.arange(T0,0,-0.5):
if(T == 0):
continue
beta = 1/(k_B*T)
for step in range(num_iterations):
# Pick a random site and calculate its local field
i,j = np.random.randint(0,N,[1,2]).flatten()
x_n = mapN[i,j]
b_n = 0
## print(i,j)
# Neighbours
ip1 = np.mod(i+1,N)
im1 = np.mod(i-1,N)
jp1 = np.mod(j+1,N)
jm1 = np.mod(j-1,N)
# Left, Right, Below, Above neighbours
neighbour_positions = np.array([[im1,j],[ip1,j],[i,jm1],[i,jp1]])
for n_i in range(4):
m_i,m_j = neighbour_positions[n_i,:].flatten()
b_n += J*mapN[m_i,m_j]
b_n += H
DeltaE=2*x_n*b_n
if(DeltaE<=0):
mapN[i,j] *= -1
elif(np.random.rand()<np.exp(-beta*DeltaE)):
mapN[i,j] *= -1
##print("-"*45)
print(mapN)
num_ups = np.sum(np.sum(mapN==1))
num_downs = N**2 - num_ups
magnetization = (num_ups-num_downs)/N**2
magnetization_vs_T = np.vstack((magnetization_vs_T,[T,magnetization]))
print("T:{:}\t|{:} up | {:} down\t|Mag: {:.5f} ".format(T,num_ups,num_downs,magnetization))
print("-"*45)
plt.matshow(mapN, interpolation="none", cmap=cmap, norm=norm,origin="upper")
plt.title("Temperature: {:}\nUp:{:d}|Down:{:d}".format(T,num_ups,num_downs))
plt.savefig("/tmp/figs/" + "T_%.3f" % (T) + ".png")
plt.show()
print(magnetization_vs_T)
plt.plot(magnetization_vs_T[:,0],magnetization_vs_T[:,1],"*-")
plt.ylim(-1.1,1.1)
plt.xlabel("T")
plt.ylabel("<M>")
#plt.savefig("/tmp/figs/" + "MeanMag" + ".png")
plt.show()
print("Finished.")
[[ 1 1 -1 -1 -1 -1 1 -1 1 1]
[-1 -1 -1 -1 1 1 1 -1 1 1]
[-1 1 -1 1 -1 1 1 -1 1 -1]
[-1 -1 -1 -1 -1 -1 -1 1 1 1]
[ 1 1 -1 1 -1 1 -1 -1 1 -1]
[ 1 -1 -1 1 -1 -1 -1 1 1 1]
[-1 1 -1 1 -1 1 1 -1 -1 1]
[ 1 -1 1 1 1 -1 1 -1 1 1]
[-1 -1 1 -1 1 1 1 1 -1 -1]
[ 1 -1 -1 -1 1 -1 -1 1 -1 1]]
49 up | 51 down
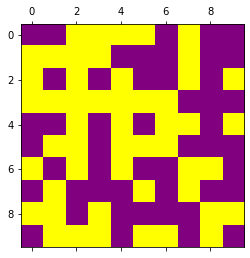
=============================================
[[ 1 1 1 1 -1 1 1 1 1 1]
[-1 1 1 1 -1 -1 -1 1 -1 -1]
[ 1 -1 -1 1 -1 1 1 1 -1 -1]
[-1 -1 -1 1 -1 -1 1 1 -1 1]
[ 1 1 1 -1 -1 -1 -1 1 1 1]
[ 1 -1 1 -1 1 -1 -1 1 -1 -1]
[-1 1 -1 -1 -1 -1 -1 -1 -1 -1]
[ 1 -1 -1 -1 1 -1 1 1 -1 1]
[-1 1 1 -1 -1 -1 -1 1 -1 1]
[ 1 -1 1 1 1 -1 -1 -1 1 -1]]
T:10.0 |47 up | 53 down |Mag: -0.06000
---------------------------------------------
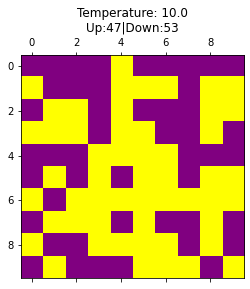
[[ 1 -1 -1 -1 -1 -1 -1 1 1 1]
[ 1 -1 -1 -1 -1 1 1 -1 -1 -1]
[-1 -1 -1 -1 1 1 -1 -1 1 -1]
[ 1 -1 1 -1 -1 1 1 1 1 1]
[ 1 1 -1 1 -1 1 -1 1 1 1]
[-1 -1 1 1 -1 1 -1 -1 -1 -1]
[ 1 1 -1 1 1 -1 -1 -1 -1 1]
[ 1 1 -1 -1 1 -1 1 1 1 -1]
[ 1 -1 1 -1 -1 -1 1 -1 -1 -1]
[ 1 -1 1 1 -1 -1 1 1 -1 -1]]
T:9.5 |46 up | 54 down |Mag: -0.08000
---------------------------------------------
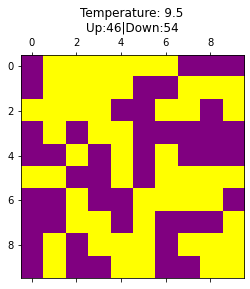
[[ 1 -1 1 1 -1 1 1 1 1 -1]
[ 1 1 -1 1 -1 -1 -1 1 1 -1]
[-1 1 -1 1 -1 -1 1 -1 1 1]
[-1 1 1 1 -1 1 1 -1 1 -1]
[-1 -1 1 -1 1 1 -1 -1 1 1]
[ 1 -1 -1 -1 1 1 1 1 -1 -1]
[ 1 -1 -1 1 1 1 1 -1 1 1]
[-1 -1 1 -1 1 -1 1 -1 -1 1]
[-1 1 1 -1 -1 -1 -1 -1 1 1]
[-1 -1 -1 -1 -1 -1 1 1 1 1]]
T:9.0 |52 up | 48 down |Mag: 0.04000
---------------------------------------------
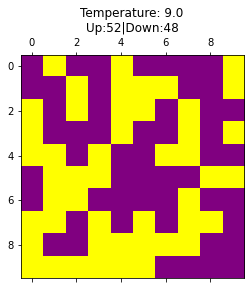
[[-1 1 -1 -1 1 -1 1 -1 -1 -1]
[-1 1 -1 1 -1 1 -1 1 1 1]
[-1 1 1 1 -1 -1 -1 1 1 1]
[-1 -1 1 1 1 1 1 -1 -1 -1]
[ 1 -1 -1 1 1 -1 1 -1 -1 -1]
[-1 1 1 1 -1 -1 1 -1 -1 -1]
[-1 -1 1 1 -1 -1 1 -1 -1 -1]
[-1 1 1 -1 1 1 1 -1 1 1]
[ 1 -1 1 1 -1 1 -1 -1 1 1]
[-1 -1 -1 -1 1 -1 -1 -1 -1 1]]
T:8.5 |46 up | 54 down |Mag: -0.08000
---------------------------------------------
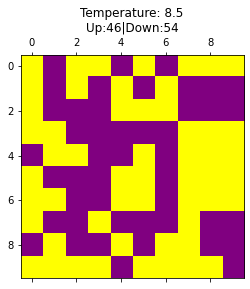
[[ 1 -1 1 -1 -1 -1 -1 1 -1 1]
[-1 -1 -1 -1 -1 1 1 1 1 1]
[ 1 1 -1 1 -1 1 1 -1 -1 1]
[ 1 1 1 1 1 1 1 -1 1 1]
[-1 -1 -1 -1 1 -1 1 -1 -1 -1]
[-1 -1 -1 1 1 -1 -1 1 -1 -1]
[ 1 -1 1 -1 1 -1 -1 1 -1 -1]
[ 1 -1 -1 -1 -1 1 -1 -1 1 -1]
[ 1 1 -1 -1 1 -1 -1 1 1 -1]
[ 1 1 -1 -1 -1 1 1 1 1 -1]]
T:8.0 |47 up | 53 down |Mag: -0.06000
---------------------------------------------
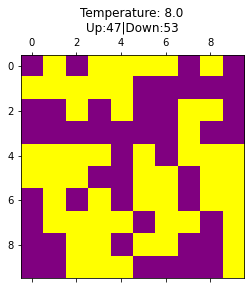
[[ 1 -1 -1 -1 -1 1 -1 -1 1 1]
[ 1 -1 -1 -1 -1 -1 -1 1 1 1]
[ 1 -1 -1 -1 -1 1 1 1 1 1]
[-1 1 1 1 1 -1 1 1 -1 1]
[-1 -1 -1 1 1 -1 -1 1 1 1]
[ 1 -1 -1 1 -1 -1 -1 -1 1 1]
[-1 -1 -1 -1 1 1 1 1 1 1]
[-1 1 1 1 1 -1 1 -1 1 1]
[ 1 1 -1 -1 -1 1 1 1 1 1]
[ 1 -1 -1 -1 -1 -1 1 1 -1 1]]
T:7.5 |54 up | 46 down |Mag: 0.08000
---------------------------------------------
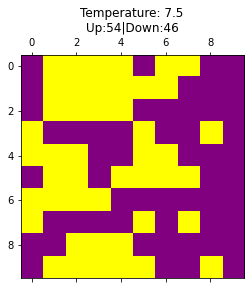
[[ 1 1 1 1 1 -1 1 1 1 1]
[ 1 -1 1 -1 -1 -1 -1 -1 -1 1]
[ 1 -1 1 -1 -1 1 -1 -1 1 1]
[ 1 1 1 -1 -1 -1 1 1 1 -1]
[ 1 -1 1 -1 -1 -1 -1 -1 1 -1]
[ 1 1 1 -1 -1 -1 -1 -1 -1 -1]
[-1 -1 1 -1 -1 -1 -1 -1 -1 -1]
[-1 -1 1 1 1 -1 -1 1 1 -1]
[-1 1 -1 -1 1 1 1 1 1 1]
[ 1 1 1 -1 1 -1 1 1 -1 1]]
T:7.0 |49 up | 51 down |Mag: -0.02000
---------------------------------------------
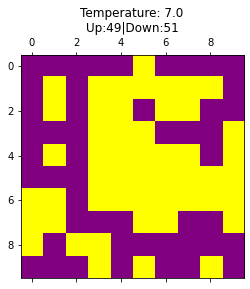
[[-1 -1 -1 -1 1 -1 1 -1 -1 -1]
[-1 -1 -1 -1 1 -1 -1 1 1 -1]
[-1 -1 -1 1 -1 -1 -1 1 1 -1]
[-1 -1 -1 -1 1 1 1 1 1 1]
[ 1 -1 -1 -1 1 1 1 1 -1 1]
[-1 -1 1 -1 1 1 -1 1 1 1]
[ 1 1 -1 -1 1 -1 -1 -1 1 1]
[ 1 -1 1 -1 -1 -1 1 1 1 1]
[-1 -1 -1 -1 -1 1 -1 1 1 -1]
[ 1 -1 -1 -1 1 -1 -1 -1 -1 -1]]
T:6.5 |42 up | 58 down |Mag: -0.16000
---------------------------------------------
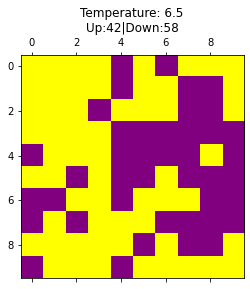
[[-1 -1 -1 -1 1 1 -1 -1 1 -1]
[ 1 1 -1 -1 1 -1 -1 1 1 -1]
[ 1 1 1 1 1 -1 -1 -1 -1 1]
[-1 1 1 1 1 -1 -1 -1 -1 1]
[ 1 1 -1 -1 -1 -1 1 1 1 1]
[ 1 1 1 -1 -1 -1 -1 1 1 1]
[-1 -1 1 1 1 -1 -1 -1 1 1]
[-1 1 1 1 1 -1 1 -1 1 1]
[-1 1 1 -1 -1 -1 1 1 1 1]
[ 1 -1 -1 -1 1 1 1 -1 -1 1]]
T:6.0 |54 up | 46 down |Mag: 0.08000
---------------------------------------------
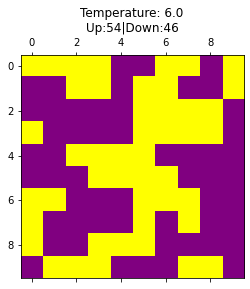
[[ 1 1 -1 -1 1 -1 -1 -1 1 1]
[ 1 1 1 1 -1 -1 -1 -1 1 -1]
[ 1 -1 -1 1 -1 1 -1 -1 1 1]
[-1 -1 -1 -1 -1 -1 -1 -1 -1 1]
[-1 1 1 1 -1 1 -1 -1 -1 -1]
[-1 1 1 1 -1 -1 1 -1 -1 1]
[ 1 1 1 1 -1 1 -1 -1 -1 -1]
[-1 -1 -1 -1 -1 1 1 1 1 1]
[-1 -1 -1 -1 -1 -1 -1 -1 1 -1]
[ 1 1 1 -1 1 1 -1 -1 1 1]]
T:5.5 |43 up | 57 down |Mag: -0.14000
---------------------------------------------
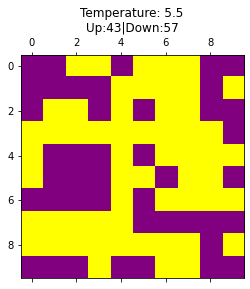
[[ 1 1 -1 -1 -1 1 -1 -1 1 -1]
[-1 -1 -1 1 1 1 -1 1 -1 -1]
[ 1 1 1 1 1 1 -1 -1 -1 1]
[ 1 1 1 1 1 1 -1 1 1 1]
[ 1 -1 -1 -1 -1 -1 -1 1 1 1]
[ 1 -1 -1 1 -1 -1 1 1 -1 -1]
[ 1 1 1 1 1 -1 -1 -1 -1 1]
[ 1 1 -1 -1 1 1 -1 1 -1 -1]
[ 1 1 -1 1 1 1 1 1 1 -1]
[ 1 1 -1 1 -1 -1 -1 1 1 1]]
T:5.0 |57 up | 43 down |Mag: 0.14000
---------------------------------------------
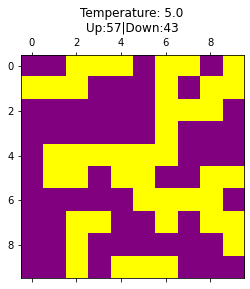
[[-1 -1 -1 -1 1 1 1 1 1 1]
[-1 -1 -1 -1 1 1 -1 -1 -1 1]
[-1 -1 1 -1 1 1 1 -1 -1 1]
[-1 1 1 -1 1 1 1 1 -1 1]
[ 1 -1 -1 -1 -1 1 1 1 -1 -1]
[ 1 -1 -1 -1 -1 -1 1 -1 -1 1]
[ 1 1 1 1 1 1 1 -1 -1 1]
[ 1 1 1 1 1 -1 -1 -1 1 1]
[ 1 -1 1 1 1 -1 -1 -1 -1 -1]
[-1 1 -1 1 1 -1 1 1 1 -1]]
T:4.5 |53 up | 47 down |Mag: 0.06000
---------------------------------------------
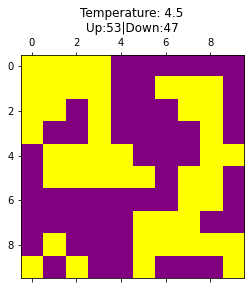
[[ 1 1 1 1 1 -1 1 1 -1 1]
[ 1 1 1 1 1 -1 -1 1 -1 1]
[ 1 1 1 1 1 1 1 1 1 1]
[-1 -1 1 -1 1 1 -1 1 1 1]
[ 1 1 1 -1 1 1 -1 1 1 1]
[-1 -1 1 -1 -1 1 -1 -1 -1 -1]
[-1 -1 -1 -1 -1 -1 1 -1 -1 -1]
[-1 -1 -1 -1 1 1 1 -1 -1 1]
[-1 -1 -1 -1 1 1 1 -1 -1 -1]
[-1 1 -1 -1 -1 -1 1 1 -1 -1]]
T:4.0 |52 up | 48 down |Mag: 0.04000
---------------------------------------------
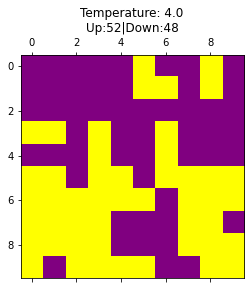
[[ 1 -1 1 1 1 1 -1 1 -1 1]
[-1 -1 1 1 -1 -1 -1 -1 -1 -1]
[ 1 -1 -1 -1 1 1 1 -1 1 1]
[-1 -1 1 1 1 1 1 1 1 -1]
[-1 -1 -1 -1 1 -1 -1 -1 -1 -1]
[-1 -1 -1 -1 1 -1 -1 -1 -1 -1]
[-1 1 1 1 1 1 -1 -1 -1 -1]
[-1 1 1 1 1 1 -1 -1 1 -1]
[ 1 1 -1 1 1 -1 -1 1 1 1]
[ 1 1 1 1 1 1 1 -1 -1 1]]
T:3.5 |50 up | 50 down |Mag: 0.00000
---------------------------------------------
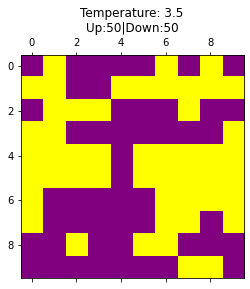
[[ 1 -1 -1 -1 -1 -1 -1 1 1 -1]
[ 1 -1 -1 -1 -1 -1 -1 1 -1 1]
[-1 -1 -1 -1 -1 -1 -1 1 1 -1]
[ 1 -1 1 -1 -1 -1 1 1 1 1]
[ 1 1 1 1 1 1 -1 1 1 -1]
[ 1 1 1 1 1 1 1 1 1 1]
[ 1 -1 1 1 1 1 1 1 1 1]
[-1 -1 1 1 1 -1 1 1 1 1]
[-1 -1 1 1 1 -1 -1 -1 -1 1]
[ 1 -1 -1 -1 -1 -1 1 1 1 -1]]
T:3.0 |56 up | 44 down |Mag: 0.12000
---------------------------------------------
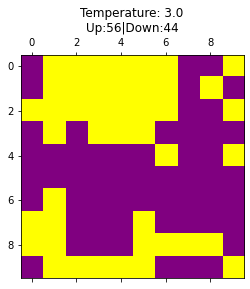
[[ 1 1 1 -1 -1 -1 1 1 1 1]
[ 1 1 1 1 -1 -1 -1 1 1 1]
[-1 -1 -1 1 -1 -1 -1 1 1 1]
[ 1 1 1 1 -1 1 1 1 1 1]
[ 1 1 1 1 -1 1 1 -1 1 1]
[ 1 1 -1 -1 -1 1 1 1 1 1]
[ 1 -1 -1 -1 -1 1 -1 1 1 1]
[ 1 1 1 -1 -1 -1 -1 1 1 1]
[ 1 1 1 1 -1 -1 -1 1 1 1]
[ 1 1 1 1 -1 -1 1 1 1 1]]
T:2.5 |68 up | 32 down |Mag: 0.36000
---------------------------------------------
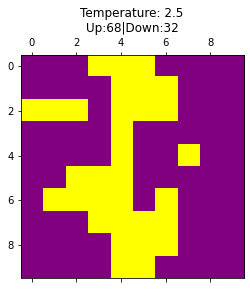
[[ 1 1 1 1 1 -1 1 1 1 1]
[ 1 1 1 1 1 1 1 1 1 1]
[-1 1 1 1 1 1 1 1 1 1]
[ 1 1 1 1 1 1 1 1 1 1]
[ 1 1 1 1 1 1 1 1 1 1]
[ 1 1 1 1 1 1 1 1 1 1]
[ 1 1 1 1 1 1 1 1 1 1]
[ 1 1 1 1 1 1 1 1 1 1]
[ 1 1 1 1 1 1 1 1 1 1]
[ 1 1 1 1 1 1 1 1 1 1]]
T:2.0 |98 up | 2 down |Mag: 0.96000
---------------------------------------------
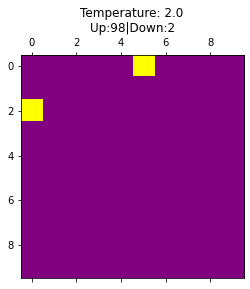
[[ 1 1 1 1 1 1 1 1 1 1]
[ 1 1 1 1 1 1 1 1 1 1]
[ 1 -1 1 1 1 1 1 1 1 1]
[ 1 1 1 1 1 1 1 1 1 1]
[ 1 1 1 1 1 1 1 1 1 1]
[ 1 1 1 1 1 1 1 1 1 1]
[ 1 1 1 1 1 1 1 1 1 1]
[ 1 1 -1 1 1 1 1 1 1 1]
[ 1 1 1 1 1 1 1 1 1 1]
[ 1 1 1 1 1 1 1 1 1 1]]
T:1.5 |98 up | 2 down |Mag: 0.96000
---------------------------------------------
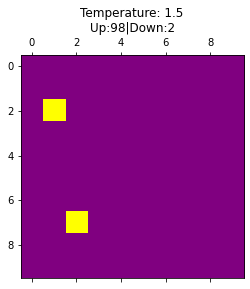
[[1 1 1 1 1 1 1 1 1 1]
[1 1 1 1 1 1 1 1 1 1]
[1 1 1 1 1 1 1 1 1 1]
[1 1 1 1 1 1 1 1 1 1]
[1 1 1 1 1 1 1 1 1 1]
[1 1 1 1 1 1 1 1 1 1]
[1 1 1 1 1 1 1 1 1 1]
[1 1 1 1 1 1 1 1 1 1]
[1 1 1 1 1 1 1 1 1 1]
[1 1 1 1 1 1 1 1 1 1]]
T:1.0 |100 up | 0 down |Mag: 1.00000
---------------------------------------------
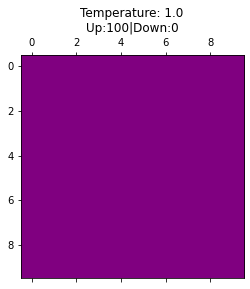
[[1 1 1 1 1 1 1 1 1 1]
[1 1 1 1 1 1 1 1 1 1]
[1 1 1 1 1 1 1 1 1 1]
[1 1 1 1 1 1 1 1 1 1]
[1 1 1 1 1 1 1 1 1 1]
[1 1 1 1 1 1 1 1 1 1]
[1 1 1 1 1 1 1 1 1 1]
[1 1 1 1 1 1 1 1 1 1]
[1 1 1 1 1 1 1 1 1 1]
[1 1 1 1 1 1 1 1 1 1]]
T:0.5 |100 up | 0 down |Mag: 1.00000
---------------------------------------------
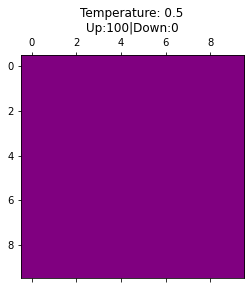
[[10. -0.06]
[ 9.5 -0.08]
[ 9. 0.04]
[ 8.5 -0.08]
[ 8. -0.06]
[ 7.5 0.08]
[ 7. -0.02]
[ 6.5 -0.16]
[ 6. 0.08]
[ 5.5 -0.14]
[ 5. 0.14]
[ 4.5 0.06]
[ 4. 0.04]
[ 3.5 0. ]
[ 3. 0.12]
[ 2.5 0.36]
[ 2. 0.96]
[ 1.5 0.96]
[ 1. 1. ]
[ 0.5 1. ]]
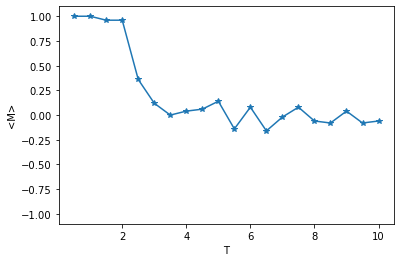
Finished.
Homework#
Show that a flip of the spin \(x_n\) of site \(n\) indeed changes the energy by:
where \(b_n\) is the local field defined as:
Appendix : Evolution of the configuration probabilities for a 2x2 Ising Grid#
A 2x2 grid system can be in one of the \(2^4=16\) configurations:
A (x1) |
B (x4) |
C (x6) |
D (x4) |
E (x1) |
---|---|---|---|---|
↑ ↑ |
↓ ↑ |
↑ ↑ |
↑ ↓ |
↓ ↓ |
↑ ↑ |
↑ ↑ |
↓ ↓ |
↓ ↓ |
↓ ↓ |
The calculated energies with respect to each spin’s interaction with its neighbours yields (taking \(J=1,\,H=0\)):
Let’s proceed by calculating the probabilites at a given temperature \(T\) (with \(k_B\) taken as \(1\)):
import numpy as np
k_B = 1
E = np.array([-6,0,2,0,-6])
multiplicity = np.array([1,4,6,4,1])
results = []
for T in np.concatenate((np.arange(300,10,-1),np.arange(10,0,-0.5))):
Probabilities = multiplicity*np.exp(-E/(k_B*T))
Z = Probabilities.sum()
Probabilities /= Z
print(T,Probabilities)
results.append([T,*Probabilities])
results = np.array(results)
300.0 [0.06376045 0.24999163 0.37249584 0.24999163 0.06376045]
299.0 [0.0637647 0.24999157 0.37248745 0.24999157 0.0637647 ]
298.0 [0.06376898 0.24999152 0.37247901 0.24999152 0.06376898]
297.0 [0.06377329 0.24999146 0.37247051 0.24999146 0.06377329]
296.0 [0.06377763 0.2499914 0.37246195 0.2499914 0.06377763]
295.0 [0.06378199 0.24999134 0.37245333 0.24999134 0.06378199]
294.0 [0.06378639 0.24999128 0.37244465 0.24999128 0.06378639]
293.0 [0.06379082 0.24999122 0.37243592 0.24999122 0.06379082]
292.0 [0.06379528 0.24999116 0.37242712 0.24999116 0.06379528]
291.0 [0.06379977 0.2499911 0.37241826 0.2499911 0.06379977]
290.0 [0.06380429 0.24999104 0.37240934 0.24999104 0.06380429]
289.0 [0.06380884 0.24999098 0.37240037 0.24999098 0.06380884]
288.0 [0.06381342 0.24999092 0.37239132 0.24999092 0.06381342]
287.0 [0.06381804 0.24999085 0.37238222 0.24999085 0.06381804]
286.0 [0.06382269 0.24999079 0.37237305 0.24999079 0.06382269]
285.0 [0.06382737 0.24999072 0.37236382 0.24999072 0.06382737]
284.0 [0.06383208 0.24999066 0.37235452 0.24999066 0.06383208]
283.0 [0.06383683 0.24999059 0.37234515 0.24999059 0.06383683]
282.0 [0.06384161 0.24999052 0.37233572 0.24999052 0.06384161]
281.0 [0.06384643 0.24999046 0.37232622 0.24999046 0.06384643]
280.0 [0.06385128 0.24999039 0.37231666 0.24999039 0.06385128]
279.0 [0.06385617 0.24999032 0.37230702 0.24999032 0.06385617]
278.0 [0.06386109 0.24999025 0.37229732 0.24999025 0.06386109]
277.0 [0.06386605 0.24999018 0.37228754 0.24999018 0.06386605]
276.0 [0.06387104 0.24999011 0.3722777 0.24999011 0.06387104]
275.0 [0.06387607 0.24999003 0.37226778 0.24999003 0.06387607]
274.0 [0.06388114 0.24998996 0.37225779 0.24998996 0.06388114]
273.0 [0.06388625 0.24998989 0.37224773 0.24998989 0.06388625]
272.0 [0.06389139 0.24998981 0.37223759 0.24998981 0.06389139]
271.0 [0.06389657 0.24998974 0.37222738 0.24998974 0.06389657]
270.0 [0.06390179 0.24998966 0.37221709 0.24998966 0.06390179]
269.0 [0.06390705 0.24998958 0.37220673 0.24998958 0.06390705]
268.0 [0.06391235 0.24998951 0.37219628 0.24998951 0.06391235]
267.0 [0.06391769 0.24998943 0.37218576 0.24998943 0.06391769]
266.0 [0.06392307 0.24998935 0.37217516 0.24998935 0.06392307]
265.0 [0.06392849 0.24998927 0.37216449 0.24998927 0.06392849]
264.0 [0.06393395 0.24998918 0.37215372 0.24998918 0.06393395]
263.0 [0.06393946 0.2499891 0.37214288 0.2499891 0.06393946]
262.0 [0.063945 0.24998902 0.37213196 0.24998902 0.063945 ]
261.0 [0.06395059 0.24998893 0.37212095 0.24998893 0.06395059]
260.0 [0.06395623 0.24998885 0.37210985 0.24998885 0.06395623]
259.0 [0.0639619 0.24998876 0.37209867 0.24998876 0.0639619 ]
258.0 [0.06396762 0.24998867 0.3720874 0.24998867 0.06396762]
257.0 [0.06397339 0.24998859 0.37207605 0.24998859 0.06397339]
256.0 [0.0639792 0.2499885 0.37206461 0.2499885 0.0639792 ]
255.0 [0.06398506 0.24998841 0.37205307 0.24998841 0.06398506]
254.0 [0.06399096 0.24998831 0.37204145 0.24998831 0.06399096]
253.0 [0.06399691 0.24998822 0.37202973 0.24998822 0.06399691]
252.0 [0.06400291 0.24998813 0.37201792 0.24998813 0.06400291]
251.0 [0.06400896 0.24998803 0.37200602 0.24998803 0.06400896]
250.0 [0.06401506 0.24998794 0.37199402 0.24998794 0.06401506]
249.0 [0.0640212 0.24998784 0.37198192 0.24998784 0.0640212 ]
248.0 [0.0640274 0.24998774 0.37196973 0.24998774 0.0640274 ]
247.0 [0.06403364 0.24998764 0.37195743 0.24998764 0.06403364]
246.0 [0.06403994 0.24998754 0.37194504 0.24998754 0.06403994]
245.0 [0.06404629 0.24998744 0.37193255 0.24998744 0.06404629]
244.0 [0.06405269 0.24998733 0.37191995 0.24998733 0.06405269]
243.0 [0.06405915 0.24998723 0.37190725 0.24998723 0.06405915]
242.0 [0.06406566 0.24998712 0.37189444 0.24998712 0.06406566]
241.0 [0.06407222 0.24998702 0.37188153 0.24998702 0.06407222]
240.0 [0.06407884 0.24998691 0.37186851 0.24998691 0.06407884]
239.0 [0.06408551 0.2499868 0.37185538 0.2499868 0.06408551]
238.0 [0.06409225 0.24998669 0.37184214 0.24998669 0.06409225]
237.0 [0.06409903 0.24998657 0.37182879 0.24998657 0.06409903]
236.0 [0.06410588 0.24998646 0.37181532 0.24998646 0.06410588]
235.0 [0.06411279 0.24998634 0.37180174 0.24998634 0.06411279]
234.0 [0.06411975 0.24998622 0.37178804 0.24998622 0.06411975]
233.0 [0.06412678 0.24998611 0.37177423 0.24998611 0.06412678]
232.0 [0.06413387 0.24998599 0.37176029 0.24998599 0.06413387]
231.0 [0.06414102 0.24998586 0.37174624 0.24998586 0.06414102]
230.0 [0.06414823 0.24998574 0.37173206 0.24998574 0.06414823]
229.0 [0.0641555 0.24998562 0.37171776 0.24998562 0.0641555 ]
228.0 [0.06416284 0.24998549 0.37170333 0.24998549 0.06416284]
227.0 [0.06417025 0.24998536 0.37168878 0.24998536 0.06417025]
226.0 [0.06417772 0.24998523 0.3716741 0.24998523 0.06417772]
225.0 [0.06418526 0.2499851 0.37165928 0.2499851 0.06418526]
224.0 [0.06419287 0.24998496 0.37164433 0.24998496 0.06419287]
223.0 [0.06420054 0.24998483 0.37162925 0.24998483 0.06420054]
222.0 [0.06420829 0.24998469 0.37161404 0.24998469 0.06420829]
221.0 [0.06421611 0.24998455 0.37159868 0.24998455 0.06421611]
220.0 [0.064224 0.24998441 0.37158319 0.24998441 0.064224 ]
219.0 [0.06423196 0.24998427 0.37156755 0.24998427 0.06423196]
218.0 [0.06423999 0.24998412 0.37155177 0.24998412 0.06423999]
217.0 [0.0642481 0.24998398 0.37153584 0.24998398 0.0642481 ]
216.0 [0.06425629 0.24998383 0.37151977 0.24998383 0.06425629]
215.0 [0.06426455 0.24998367 0.37150354 0.24998367 0.06426455]
214.0 [0.0642729 0.24998352 0.37148716 0.24998352 0.0642729 ]
213.0 [0.06428132 0.24998337 0.37147063 0.24998337 0.06428132]
212.0 [0.06428982 0.24998321 0.37145395 0.24998321 0.06428982]
211.0 [0.0642984 0.24998305 0.3714371 0.24998305 0.0642984 ]
210.0 [0.06430707 0.24998289 0.3714201 0.24998289 0.06430707]
209.0 [0.06431582 0.24998272 0.37140293 0.24998272 0.06431582]
208.0 [0.06432465 0.24998255 0.37138559 0.24998255 0.06432465]
207.0 [0.06433357 0.24998238 0.37136809 0.24998238 0.06433357]
206.0 [0.06434258 0.24998221 0.37135042 0.24998221 0.06434258]
205.0 [0.06435168 0.24998204 0.37133257 0.24998204 0.06435168]
204.0 [0.06436086 0.24998186 0.37131455 0.24998186 0.06436086]
203.0 [0.06437014 0.24998168 0.37129635 0.24998168 0.06437014]
202.0 [0.06437952 0.2499815 0.37127797 0.2499815 0.06437952]
201.0 [0.06438898 0.24998131 0.37125941 0.24998131 0.06438898]
200.0 [0.06439855 0.24998113 0.37124066 0.24998113 0.06439855]
199.0 [0.06440821 0.24998093 0.37122172 0.24998093 0.06440821]
198.0 [0.06441796 0.24998074 0.37120259 0.24998074 0.06441796]
197.0 [0.06442782 0.24998054 0.37118326 0.24998054 0.06442782]
196.0 [0.06443778 0.24998034 0.37116374 0.24998034 0.06443778]
195.0 [0.06444785 0.24998014 0.37114402 0.24998014 0.06444785]
194.0 [0.06445802 0.24997994 0.37112409 0.24997994 0.06445802]
193.0 [0.06446829 0.24997973 0.37110396 0.24997973 0.06446829]
192.0 [0.06447868 0.24997951 0.37108361 0.24997951 0.06447868]
191.0 [0.06448917 0.2499793 0.37106306 0.2499793 0.06448917]
190.0 [0.06449978 0.24997908 0.37104228 0.24997908 0.06449978]
189.0 [0.0645105 0.24997886 0.37102129 0.24997886 0.0645105 ]
188.0 [0.06452134 0.24997863 0.37100007 0.24997863 0.06452134]
187.0 [0.06453229 0.2499784 0.37097862 0.2499784 0.06453229]
186.0 [0.06454336 0.24997817 0.37095694 0.24997817 0.06454336]
185.0 [0.06455456 0.24997793 0.37093503 0.24997793 0.06455456]
184.0 [0.06456587 0.24997769 0.37091288 0.24997769 0.06456587]
183.0 [0.06457732 0.24997744 0.37089048 0.24997744 0.06457732]
182.0 [0.06458889 0.24997719 0.37086784 0.24997719 0.06458889]
181.0 [0.06460059 0.24997694 0.37084495 0.24997694 0.06460059]
180.0 [0.06461242 0.24997668 0.3708218 0.24997668 0.06461242]
179.0 [0.06462438 0.24997642 0.3707984 0.24997642 0.06462438]
178.0 [0.06463648 0.24997615 0.37077473 0.24997615 0.06463648]
177.0 [0.06464872 0.24997588 0.37075079 0.24997588 0.06464872]
176.0 [0.06466111 0.2499756 0.37072658 0.2499756 0.06466111]
175.0 [0.06467363 0.24997532 0.37070209 0.24997532 0.06467363]
174.0 [0.0646863 0.24997504 0.37067732 0.24997504 0.0646863 ]
173.0 [0.06469912 0.24997475 0.37065226 0.24997475 0.06469912]
172.0 [0.06471209 0.24997445 0.37062691 0.24997445 0.06471209]
171.0 [0.06472522 0.24997415 0.37060126 0.24997415 0.06472522]
170.0 [0.0647385 0.24997385 0.37057531 0.24997385 0.0647385 ]
169.0 [0.06475194 0.24997353 0.37054905 0.24997353 0.06475194]
168.0 [0.06476554 0.24997322 0.37052248 0.24997322 0.06476554]
167.0 [0.06477931 0.24997289 0.37049559 0.24997289 0.06477931]
166.0 [0.06479325 0.24997256 0.37046838 0.24997256 0.06479325]
165.0 [0.06480736 0.24997223 0.37044083 0.24997223 0.06480736]
164.0 [0.06482164 0.24997189 0.37041295 0.24997189 0.06482164]
163.0 [0.0648361 0.24997154 0.37038472 0.24997154 0.0648361 ]
162.0 [0.06485074 0.24997119 0.37035614 0.24997119 0.06485074]
161.0 [0.06486557 0.24997083 0.37032721 0.24997083 0.06486557]
160.0 [0.06488058 0.24997046 0.37029792 0.24997046 0.06488058]
159.0 [0.06489579 0.24997009 0.37026825 0.24997009 0.06489579]
158.0 [0.06491119 0.2499697 0.37023821 0.2499697 0.06491119]
157.0 [0.06492679 0.24996932 0.37020778 0.24996932 0.06492679]
156.0 [0.0649426 0.24996892 0.37017697 0.24996892 0.0649426 ]
155.0 [0.06495861 0.24996851 0.37014575 0.24996851 0.06495861]
154.0 [0.06497483 0.2499681 0.37011413 0.2499681 0.06497483]
153.0 [0.06499127 0.24996768 0.37008209 0.24996768 0.06499127]
152.0 [0.06500793 0.24996725 0.37004963 0.24996725 0.06500793]
151.0 [0.06502481 0.24996682 0.37001674 0.24996682 0.06502481]
150.0 [0.06504192 0.24996637 0.36998341 0.24996637 0.06504192]
149.0 [0.06505927 0.24996592 0.36994963 0.24996592 0.06505927]
148.0 [0.06507685 0.24996545 0.36991539 0.24996545 0.06507685]
147.0 [0.06509468 0.24996498 0.36988069 0.24996498 0.06509468]
146.0 [0.06511275 0.2499645 0.36984551 0.2499645 0.06511275]
145.0 [0.06513108 0.249964 0.36980984 0.249964 0.06513108]
144.0 [0.06514967 0.2499635 0.36977367 0.2499635 0.06514967]
143.0 [0.06516852 0.24996298 0.369737 0.24996298 0.06516852]
142.0 [0.06518764 0.24996246 0.3696998 0.24996246 0.06518764]
141.0 [0.06520704 0.24996192 0.36966208 0.24996192 0.06520704]
140.0 [0.06522672 0.24996137 0.36962382 0.24996137 0.06522672]
139.0 [0.06524669 0.24996081 0.36958501 0.24996081 0.06524669]
138.0 [0.06526695 0.24996024 0.36954563 0.24996024 0.06526695]
137.0 [0.06528751 0.24995965 0.36950567 0.24995965 0.06528751]
136.0 [0.06530838 0.24995905 0.36946512 0.24995905 0.06530838]
135.0 [0.06532957 0.24995844 0.36942398 0.24995844 0.06532957]
134.0 [0.06535108 0.24995782 0.36938221 0.24995782 0.06535108]
133.0 [0.06537292 0.24995718 0.36933981 0.24995718 0.06537292]
132.0 [0.06539509 0.24995652 0.36929677 0.24995652 0.06539509]
131.0 [0.06541761 0.24995585 0.36925307 0.24995585 0.06541761]
130.0 [0.06544048 0.24995517 0.3692087 0.24995517 0.06544048]
129.0 [0.06546372 0.24995447 0.36916363 0.24995447 0.06546372]
128.0 [0.06548732 0.24995375 0.36911786 0.24995375 0.06548732]
127.0 [0.0655113 0.24995301 0.36907137 0.24995301 0.0655113 ]
126.0 [0.06553567 0.24995226 0.36902413 0.24995226 0.06553567]
125.0 [0.06556044 0.24995149 0.36897614 0.24995149 0.06556044]
124.0 [0.06558562 0.2499507 0.36892736 0.2499507 0.06558562]
123.0 [0.06561121 0.24994989 0.36887779 0.24994989 0.06561121]
122.0 [0.06563723 0.24994906 0.36882741 0.24994906 0.06563723]
121.0 [0.06566369 0.24994821 0.36877619 0.24994821 0.06566369]
120.0 [0.0656906 0.24994734 0.36872411 0.24994734 0.0656906 ]
119.0 [0.06571798 0.24994645 0.36867116 0.24994645 0.06571798]
118.0 [0.06574582 0.24994553 0.3686173 0.24994553 0.06574582]
117.0 [0.06577415 0.24994459 0.36856251 0.24994459 0.06577415]
116.0 [0.06580298 0.24994363 0.36850678 0.24994363 0.06580298]
115.0 [0.06583233 0.24994263 0.36845008 0.24994263 0.06583233]
114.0 [0.06586219 0.24994162 0.36839238 0.24994162 0.06586219]
113.0 [0.0658926 0.24994057 0.36833365 0.24994057 0.0658926 ]
112.0 [0.06592356 0.2499395 0.36827387 0.2499395 0.06592356]
111.0 [0.0659551 0.2499384 0.368213 0.2499384 0.0659551]
110.0 [0.06598722 0.24993727 0.36815103 0.24993727 0.06598722]
109.0 [0.06601994 0.24993611 0.36808791 0.24993611 0.06601994]
108.0 [0.06605328 0.24993491 0.36802362 0.24993491 0.06605328]
107.0 [0.06608726 0.24993368 0.36795812 0.24993368 0.06608726]
106.0 [0.0661219 0.24993241 0.36789138 0.24993241 0.0661219 ]
105.0 [0.06615721 0.24993111 0.36782336 0.24993111 0.06615721]
104.0 [0.06619321 0.24992977 0.36775403 0.24992977 0.06619321]
103.0 [0.06622993 0.24992839 0.36768334 0.24992839 0.06622993]
102.0 [0.06626739 0.24992698 0.36761127 0.24992698 0.06626739]
101.0 [0.06630561 0.24992551 0.36753776 0.24992551 0.06630561]
100.0 [0.06634461 0.24992401 0.36746277 0.24992401 0.06634461]
99.0 [0.06638442 0.24992245 0.36738626 0.24992245 0.06638442]
98.0 [0.06642506 0.24992085 0.36730818 0.24992085 0.06642506]
97.0 [0.06646656 0.2499192 0.36722848 0.2499192 0.06646656]
96.0 [0.06650895 0.2499175 0.36714711 0.2499175 0.06650895]
95.0 [0.06655225 0.24991574 0.36706403 0.24991574 0.06655225]
94.0 [0.0665965 0.24991392 0.36697916 0.24991392 0.0665965 ]
93.0 [0.06664172 0.24991205 0.36689246 0.24991205 0.06664172]
92.0 [0.06668795 0.24991011 0.36680387 0.24991011 0.06668795]
91.0 [0.06673523 0.24990811 0.36671332 0.24990811 0.06673523]
90.0 [0.06678359 0.24990604 0.36662074 0.24990604 0.06678359]
89.0 [0.06683306 0.24990391 0.36652607 0.24990391 0.06683306]
88.0 [0.06688369 0.24990169 0.36642924 0.24990169 0.06688369]
87.0 [0.06693551 0.2498994 0.36633018 0.2498994 0.06693551]
86.0 [0.06698857 0.24989703 0.36622879 0.24989703 0.06698857]
85.0 [0.06704292 0.24989458 0.36612501 0.24989458 0.06704292]
84.0 [0.0670986 0.24989203 0.36601874 0.24989203 0.0670986 ]
83.0 [0.06715566 0.24988939 0.3659099 0.24988939 0.06715566]
82.0 [0.06721415 0.24988666 0.36579838 0.24988666 0.06721415]
81.0 [0.06727413 0.24988382 0.3656841 0.24988382 0.06727413]
80.0 [0.06733566 0.24988087 0.36556694 0.24988087 0.06733566]
79.0 [0.06739879 0.24987781 0.3654468 0.24987781 0.06739879]
78.0 [0.06746359 0.24987463 0.36532355 0.24987463 0.06746359]
77.0 [0.06753013 0.24987133 0.36519709 0.24987133 0.06753013]
76.0 [0.06759847 0.24986789 0.36506728 0.24986789 0.06759847]
75.0 [0.06766869 0.24986431 0.36493398 0.24986431 0.06766869]
74.0 [0.06774088 0.24986059 0.36479706 0.24986059 0.06774088]
73.0 [0.06781511 0.24985671 0.36465636 0.24985671 0.06781511]
72.0 [0.06789147 0.24985267 0.36451173 0.24985267 0.06789147]
71.0 [0.06797005 0.24984845 0.364363 0.24984845 0.06797005]
70.0 [0.06805096 0.24984405 0.36420999 0.24984405 0.06805096]
69.0 [0.06813429 0.24983945 0.36405251 0.24983945 0.06813429]
68.0 [0.06822017 0.24983465 0.36389037 0.24983465 0.06822017]
67.0 [0.0683087 0.24982963 0.36372335 0.24982963 0.0683087 ]
66.0 [0.06840001 0.24982437 0.36355124 0.24982437 0.06840001]
65.0 [0.06849423 0.24981888 0.36337379 0.24981888 0.06849423]
64.0 [0.06859151 0.24981311 0.36319076 0.24981311 0.06859151]
63.0 [0.06869199 0.24980707 0.36300187 0.24980707 0.06869199]
62.0 [0.06879585 0.24980073 0.36280684 0.24980073 0.06879585]
61.0 [0.06890324 0.24979408 0.36260537 0.24979408 0.06890324]
60.0 [0.06901435 0.24978708 0.36239713 0.24978708 0.06901435]
59.0 [0.06912939 0.24977972 0.36218177 0.24977972 0.06912939]
58.0 [0.06924856 0.24977198 0.36195893 0.24977198 0.06924856]
57.0 [0.06937208 0.24976381 0.36172821 0.24976381 0.06937208]
56.0 [0.06950021 0.24975521 0.36148917 0.24975521 0.06950021]
55.0 [0.0696332 0.24974612 0.36124137 0.24974612 0.0696332 ]
54.0 [0.06977133 0.24973652 0.36098431 0.24973652 0.06977133]
53.0 [0.06991491 0.24972636 0.36071747 0.24972636 0.06991491]
52.0 [0.07006427 0.2497156 0.36044026 0.2497156 0.07006427]
51.0 [0.07021976 0.2497042 0.36015209 0.2497042 0.07021976]
50.0 [0.07038176 0.24969209 0.35985229 0.24969209 0.07038176]
49.0 [0.0705507 0.24967923 0.35954013 0.24967923 0.0705507 ]
48.0 [0.07072703 0.24966555 0.35921484 0.24966555 0.07072703]
47.0 [0.07091125 0.24965097 0.35887557 0.24965097 0.07091125]
46.0 [0.07110388 0.24963542 0.3585214 0.24963542 0.07110388]
45.0 [0.07130553 0.2496188 0.35815133 0.2496188 0.07130553]
44.0 [0.07151684 0.24960102 0.35776427 0.24960102 0.07151684]
43.0 [0.07173853 0.24958197 0.357359 0.24958197 0.07173853]
42.0 [0.07197136 0.24956152 0.35693423 0.24956152 0.07197136]
41.0 [0.07221621 0.24953954 0.35648851 0.24953954 0.07221621]
40.0 [0.07247402 0.24951586 0.35602024 0.24951586 0.07247402]
39.0 [0.07274585 0.24949031 0.35552769 0.24949031 0.07274585]
38.0 [0.07303288 0.24946268 0.35500889 0.24946268 0.07303288]
37.0 [0.0733364 0.24943274 0.35446172 0.24943274 0.0733364 ]
36.0 [0.07365789 0.24940023 0.35388376 0.24940023 0.07365789]
35.0 [0.07399898 0.24936484 0.35327236 0.24936484 0.07399898]
34.0 [0.07436151 0.24932623 0.35262452 0.24932623 0.07436151]
33.0 [0.07474756 0.249284 0.35193688 0.249284 0.07474756]
32.0 [0.0751595 0.24923766 0.35120567 0.24923766 0.0751595 ]
31.0 [0.07560001 0.24918668 0.35042662 0.24918668 0.07560001]
30.0 [0.07607215 0.24913042 0.34959487 0.24913042 0.07607215]
29.0 [0.07657944 0.24906811 0.3487049 0.24906811 0.07657944]
28.0 [0.07712594 0.24899887 0.34775037 0.24899887 0.07712594]
27.0 [0.07771637 0.24892162 0.34672401 0.24892162 0.07771637]
26.0 [0.07835621 0.24883509 0.34561741 0.24883509 0.07835621]
25.0 [0.0790519 0.24873771 0.34442077 0.24873771 0.0790519 ]
24.0 [0.07981104 0.24862762 0.34312268 0.24862762 0.07981104]
23.0 [0.08064268 0.24850249 0.34170966 0.24850249 0.08064268]
22.0 [0.08155764 0.24835946 0.3401658 0.24835946 0.08155764]
21.0 [0.08256901 0.24819495 0.33847206 0.24819495 0.08256901]
20.0 [0.08369275 0.24800446 0.33660557 0.24800446 0.08369275]
19.0 [0.08494851 0.24778221 0.33453856 0.24778221 0.08494851]
18.0 [0.08636076 0.24752076 0.33223696 0.24752076 0.08636076]
17.0 [0.08796037 0.24721033 0.3296586 0.24721033 0.08796037]
16.0 [0.08978677 0.24683794 0.32675058 0.24683794 0.08978677]
15.0 [0.09189118 0.24638599 0.32344567 0.24638599 0.09189118]
14.0 [0.09434121 0.24583021 0.31965716 0.24583021 0.09434121]
13.0 [0.097228 0.24513635 0.3152713 0.24513635 0.097228 ]
12.0 [0.10067707 0.24425493 0.310136 0.24425493 0.10067707]
11.0 [0.10486603 0.24311229 0.30404337 0.24311229 0.10486603]
10.0 [0.11005378 0.24159517 0.2967021 0.24159517 0.11005378]
9.5 [0.11313717 0.24064346 0.29243874 0.24064346 0.11313717]
9.0 [0.11663153 0.2395225 0.28769194 0.2395225 0.11663153]
8.5 [0.12062154 0.23819028 0.28237637 0.23819028 0.12062154]
8.0 [0.12521586 0.23659113 0.27638603 0.23659113 0.12521586]
7.5 [0.1305559 0.23465018 0.26958784 0.23465018 0.1305559 ]
7.0 [0.13682844 0.2322651 0.26181292 0.2322651 0.13682844]
6.5 [0.14428422 0.22929344 0.25284468 0.22929344 0.14428422]
6.0 [0.15326567 0.22553316 0.24240235 0.22553316 0.15326567]
5.5 [0.16424874 0.22069182 0.23011887 0.22069182 0.16424874]
5.0 [0.17790641 0.21433753 0.21551211 0.21433753 0.17790641]
4.5 [0.19520357 0.20582041 0.19795202 0.20582041 0.19520357]
4.0 [0.21753067 0.19415061 0.17663745 0.19415061 0.21753067]
3.5 [0.24685587 0.17782738 0.15063351 0.17782738 0.24685587]
3.0 [0.28574833 0.15468733 0.11912868 0.15468733 0.28574833]
2.5 [0.33666442 0.12216603 0.0823391 0.12216603 0.33666442]
2.0 [0.39869382 0.07939919 0.04381399 0.07939919 0.39869382]
1.5 [0.45966596 0.0336763 0.01331547 0.0336763 0.45966596]
1.0 [0.4945983 0.00490395 0.00099552 0.00490395 0.4945983 ]
0.5 [4.99987543e-01 1.22881186e-05 3.37597113e-07 1.22881186e-05
4.99987543e-01]
import matplotlib.pyplot as plt
fig=plt.figure(figsize=(12,8), dpi= 100, facecolor='w', edgecolor='k')
plt.subplot(2,3,1)
plt.plot(results[:,0],results[:,1],".b")
plt.legend(["$P_A$"],loc="right")
plt.xlabel("T")
plt.subplot(2,3,2)
plt.plot(results[:,0],results[:,2],".g")
plt.legend(["$P_B$"],loc="right")
plt.xlabel("T")
plt.subplot(2,3,3)
plt.plot(results[:,0],results[:,3],".r")
plt.legend(["$P_C$"],loc="right")
plt.xlabel("T")
plt.subplot(2,3,5)
plt.plot(results[:,0],results[:,4],".g")
plt.legend(["$P_D$"],loc="right")
plt.xlabel("T")
plt.subplot(2,3,4)
plt.plot(results[:,0],results[:,5],".b")
plt.legend(["$P_E$"],loc="right")
plt.xlabel("T")
plt.subplot(2,3,6)
plt.plot(results[-20:,0],results[-20:,1],".-b")
plt.plot(results[-20:,0],results[-20:,2],".-g")
plt.plot(results[-20:,0],results[-20:,3],".-r")
plt.legend(["$P_A$","$P_B$","$P_C$"])
plt.xlabel("T")
plt.show()
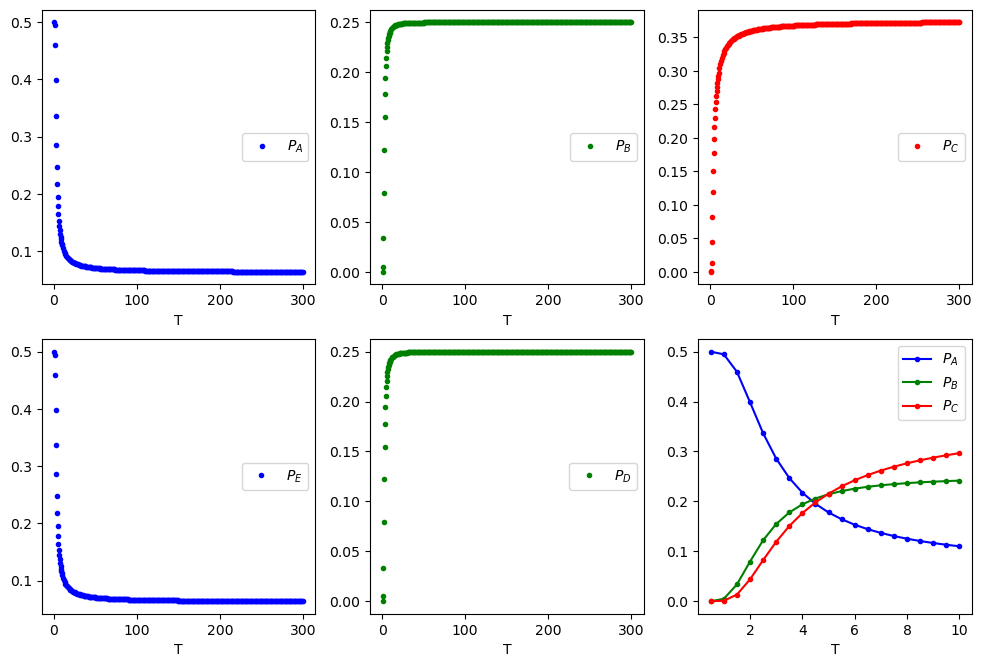
External link(s)#
Dr. David Boyce - Magnetic ordering https://twitter.com/DrDavidBoyce/status/1176921195507310593
Marco Koschny - Magnetic Domain Movement and Wall Motion (1, 2)
Andrea Benassi - Labyrinthine magnetic domains (small stray field)